Arduino Clap Switch: Controlling home appliances with a double clap!
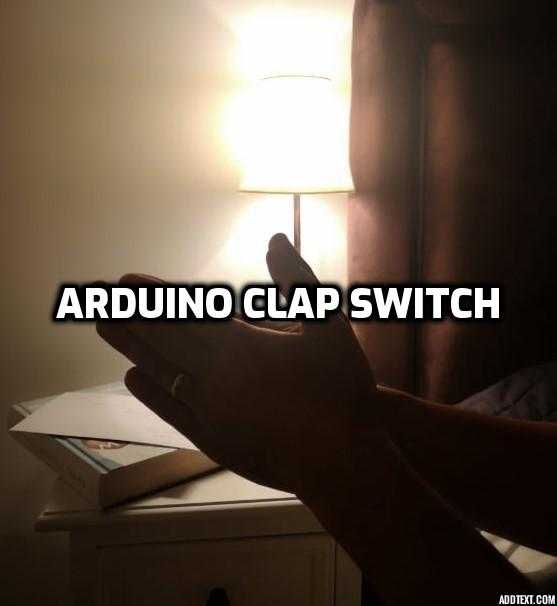
In this project, we're going to build a simple device to control lights or any other electrical appliance with a double clap.
Essentially what we're be building today is an extension cord with an attached clap switch. So instead of using a boring ON/OFF button, we'll use sound to turn things on and off and so, you can, basically, connect anything you want to it.
How does it work ?
The main idea here is to use an Arduino controlled relay that will open and close the circuit of an extension cord. If you're wondering what a relay is, you can think of it as an electromechanical switch. Heres how it works.
Inside our relay, there's a small electromagnet. When this electromagnet is supplied with a power source (e.g 5V from our Arduino) it attracts the moving part called armature. The armature then connects the contacts inside the relay and closes the external circuit.
In this context, the external circuit is the circuit of an extension cord.
Here I described how the Normally Open (NO) relay works, but most relays can be used as Normally Closed(NC) as well. NC means that the external circuit will be closed until the relay is activated. Now in our case using NC would be impractical, because a constant current should be supplied from the Arduino just to keep the lights off for example.
Now to register our claps, we will use a sound sensor that converts sound waves to electrical signal. The sound sensor we're using for this project has a patentiometer that is used to adjust the sensitivity of the sensor. This allows us to set a threshold at which the electrical signal becomes a digital LOW signal.
Finally this signal is going to be used by the Arduino to determine whether the relay should be activated or deactivated.
Warning.
In this project we'll be working with voltages that can literally kill you. So be EXTRA careful when following this tutorial. Make sure you disconnect the extension cord from the electric socket when wiring, soldering or programming your Arduino. Again I bear no responsibility if anything bad happens to you so proceed only if you feel like you're ready for this project.
The wiring.
Shopping time!
Right, so for this project we'll need:
-
RobotDyn Arduino NANO V3 (Arduino clone with micro USB but you can use any Arduino you have) ~ 3$
-
Sound Detection Sensor Module ~ 0.50$
-
3.3V/5V/12V/24V Low Level Trigger 1 Channel Relay Module (pick 5V)
Now if you're making a permanent project like me, you will also need :
-
5V 700mA 3.5W AC 220v to 5v DC step down Transformer power supply module (to supply 5V for Arduino) - ~1$.
-
Project Box (I used this one 125x80x32mm) - ~2.50$.
Also some wires, shrinkable plastic tubes for insulation and an extension cord that you can get in any local hardware store.
This is how our device looks schematatically.
Now if you prefer building this project on a breadboard first, it should look something like this:
Assembling the device.
Alright, so we'll start by removing a small part of the plastic sheath from the extension cord. If you're from Europe, you will probably see these 3 wires.
The blue wire is Neutral (N).
The brown one is Line(L).
The green-yellow is Protective Earth(PE).
Check this link if you're from another part of the world. https://www.allaboutcircuits.com/textbook/reference/chpt-2/wiring-color-codes-infographic/
So It's the N and L that interests us here.
Remove some of the insulation from the N. Make sure you don't damage the wire itself.
Completely cut off the L and remove some of insulation from both sides of the wire.
Leave PE as is.
Its important that you do it in the way I described for safety reasons. Meaning we're switchign exactly the Line(L) and not the Neutral (N) .
Now we have to solder an external wire to N which should go to the N terminal of the AC-DC converter and we'll use our relay's Common (C) terminal to connect L from mains with the AC-DC module's L terminal.
It should be clear by looking at the picture below.
The other end of the L wire should go to Normally Open(NO) terminal of the relay .
Assembling the rest from here should be straight forward. I used a small piece of stripboard to make all the required connections, but it can be done without it.
Leave the comment if you need any further explaining. Here's how it looks in my project box with everything connected according to to the schematic.
The Code
Now that we have everything in place, time to program our little device. Make sure you disconnected your extension cord from the power socket. Connect your Arduino to the PC, open the Arduino IDE and upload the code below:
int sensor = 2;
int relay = 4;
int clap = 0;
long detection_range_start = 0;
long detection_range = 0;
boolean relay_activated = false;
void setup() {
// put your setup code here, to run once:
pinMode(sensor, INPUT);
pinMode(relay, OUTPUT);
}
void loop() {
int status_sensor = digitalRead(sensor);
if (status_sensor == 0)
{
if (clap == 0)
{
detection_range_start = detection_range = millis();
clap++;
}
else if (clap > 0 && millis() - detection_range >= 50)
{
detection_range = millis();
clap++;
}
}
if (millis() - detection_range_start >= 400)
{
if (clap == 2)
{
if (!relay_activated)
{
relay_activated = true;
digitalWrite(relay, HIGH);
}
else if (relay_activated)
{
relay_activated = false;
digitalWrite(relay, LOW);
}
}
clap = 0;
}
}
Final remarks
Now you can disconnect the USB cable from Arduino, connect the extension cord to the socket and test the device. The only thing left is to actually calibrate our device. As I mentioned in the beginning it is done by adjusting the potentiometer on the sensor module. Play with it to find the perfect position.
Thanks for reading, leave your comments below and good luck on your projects!